Secure Login with Multi-Factor Authentication (MFA) in Provar
This article provides information for users who want to use Multi-Factor Authentication (MFA) to securely log in to applications. Users can use this feature to launch apps and create Provar scripts with enhanced security features.
The feature allows support for various TOTP providers like SF Authenticator, Google Authenticator, and more. The established connection can be used in all execution modes, including Run, Debug, and Test Builder while maintaining the session. The Auth Handler page object file should manage MFA TOTP scenarios, including TOTP generation and code entry on the login page.
Note: The user must enter all of the information in the Auth Handler Page Object file just once. The login sequence and success condition must be maintained by the user in the Auth Handler Page object file. Some key points to note for the secret key:
- If the application provides the key in small letters then the user needs to convert it into capital letters.
- If the application provides the key number of characters that are not divisible by 8. then the user needs to add the ‘=‘ sign to make it divisible by 8.
Follow these steps to use MFA with Provar.

1. Create UI Connection by selecting the MFA checkbox.
2. Provide all required details along with the Auth Handler file and security key.
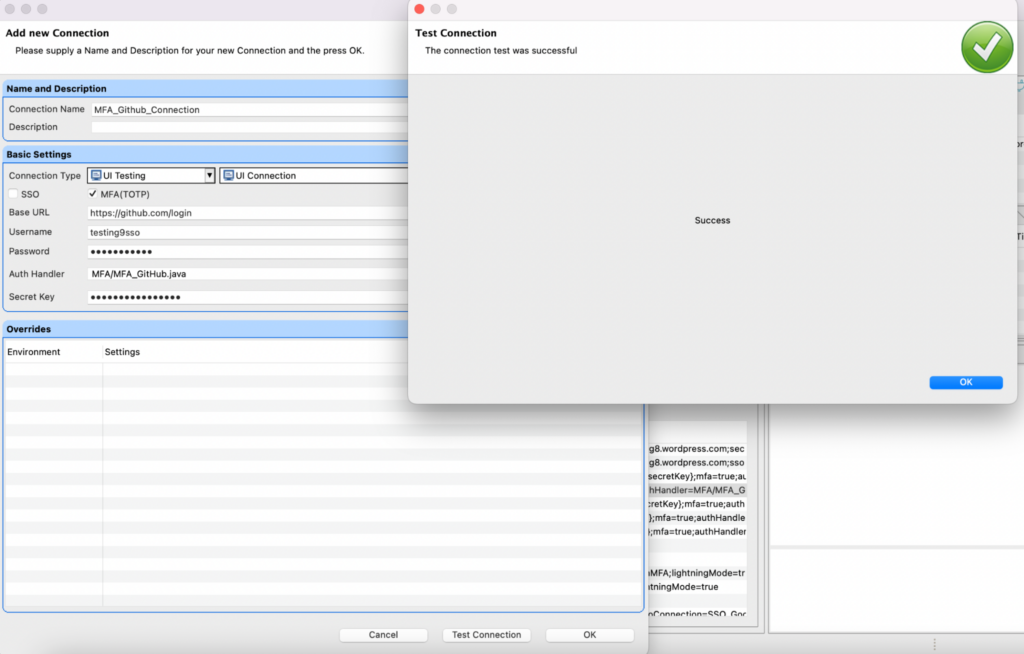
3. Verify connection using Test Connection and Click Ok
Provar’s increased security capability for logging into applications with MFA allows users to write Provar scripts in a secure and flexible manner. Secure login processes can be seamlessly integrated into users’ testing workflows.
Sample JAVA file
package pageobjects.Sample.SSO.SSOwithMFA; import java.util.Map; import org.openqa.selenium.By; import org.openqa.selenium.NoSuchElementException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import com.provar.core.model.base.api.IRuntimeConfiguration; import com.provar.core.testapi.ILoginPage; import com.provar.core.testapi.ILoginResult; import com.provar.core.testapi.annotations.Page; import com.provar.core.testapi.java.UiLoginResultImpl; @Page(title = "MFA_Login", connection = "Connection Name") public class MFA_Login implements ILoginPage { @Override public ILoginResult doLogin(IRuntimeConfiguration runtimeConfiguration, WebDriver driver, Map<String, String> credentials) { // Connection 'SSO Username' and 'SSO Password' stored in userName and password // variables String userName = credentials.get(CREDENTIAL_USER); String password = credentials.get(CREDENTIAL_PASSWORD); // User can put wait here to load the page // Check if the user is already logged in if (isLoggedIn(driver)) { return new UiLoginResultImpl(true, driver); } else { // Enter user secret details to login and submit driver.findElement(By.xpath("<Xpath for username field>")).sendKeys(userName); driver.findElement(By.xpath("<Xpath for password field>")).sendKeys(password); driver.findElement(By.xpath("<Xpath for submit/login button>")).click(); // User can put wait here to load the page (Added 2 second wait) waitForPageToLoad(2000); // Check if user navigate to successfully on opt page(if this is true it means // user still on login page). if (isInvalidLoginCredentials(driver)) { return new UiLoginResultImpl(false, "Invalid login credentials", driver); } // Get Generated OTP and enter in otp in the field int count = 0; WebElement otpElement = driver.findElement(By.xpath("<Xpath of otp field>")); while (count < 2) { try { String otp = generateTOTP(credentials); otpElement.clear(); otpElement.sendKeys(otp); driver.findElement(By.xpath("<Xpath of Verify button>")).click(); otpElement = driver.findElement(By.xpath("<Xpath of otp field>")); count++; } catch (Exception e) { break; } } // Check again if user entered correct OTP and logged in successfully boolean loginSuccess = isLoggedIn(driver); if (loginSuccess) { return new UiLoginResultImpl(true, "Login Successful.", driver); } else { return new UiLoginResultImpl(false, "Login failed : Please check your credentials.", driver); } } } private boolean isLoggedIn(WebDriver driver) { try { driver.findElement(By.xpath("<Xpath for field to check user is logged in>")); } catch (NoSuchElementException ex) { return false; } return true; } private static void waitForPageToLoad(long time) { try { Thread.sleep(time); } catch (InterruptedException e) { e.printStackTrace(); } } private boolean isInvalidLoginCredentials(WebDriver driver) { try { driver.findElement(By.xpath("<Xpath for field available in login page(like: Password field Xpath)")); } catch (NoSuchElementException ex) { return false; } return true; } }
- Provar Automation
- System Requirements
- Browser and Driver Recommendations
- Installing Provar Automation
- Updating Provar Automation
- Licensing Provar
- Granting Org Permissions to Provar Automation
- Optimizing Org and Connection Metadata Processing in Provar
- Using Provar Automation
- Understanding Provar’s Use of AI Service for Test Automation
- Provar Automation
- Creating a New Test Project
- Import Test Project from a File
- Import Test Project from a Remote Repository
- Import Test Project from Local Repository
- Commit a Local Test Project to Source Control
- Salesforce API Testing
- Behavior-Driven Development
- Consolidating Multiple Test Execution Reports
- Creating Test Cases
- Custom Table Mapping
- Functions
- Debugging Tests
- Defining a Namespace Prefix on a Connection
- Defining Proxy Settings
- Environment Management
- Exporting Test Cases into a PDF
- Exporting Test Projects
- Japanese Language Support
- Override Auto-Retry for Test Step
- Customize Browser Driver Location
- Mapping and Executing the Lightning Article Editor in Provar
- Managing Test Steps
- Namespace Org Testing
- NitroX
- Provar Test Builder
- ProvarDX
- Refresh and Recompile
- Reintroduction of CLI License Check
- Reload Org Cache
- Reporting
- Running Tests
- Searching Provar with Find Usages
- Secrets Management and Encryption
- Setup and Teardown Test Cases
- Tags and Service Level Agreements (SLAs)
- Test Cycles
- Test Data Generation
- Test Plans
- Testing Browser – Chrome Headless
- Testing Browser Options
- Tooltip Testing
- Using the Test Palette
- Using Custom APIs
- Callable Tests
- Data-Driven Testing
- Page Objects
- Block Locator Strategies
- Introduction to XPaths
- Creating an XPath
- JavaScript Locator Support
- Label Locator Strategies
- Maintaining Page Objects
- Mapping Non-Salesforce fields
- Page Object Operations
- ProvarX™
- Refresh and Reselect Field Locators in Test Builder
- Using Java Method Annotations for Custom Objects
- Applications Testing
- Database Testing
- Document Testing
- Email Testing
- Email Testing in Automation
- Email Testing Examples
- Gmail Connection in Automation with App Password
- App Configuration for Microsoft Connection in MS Portal for OAuth 2.0
- OAuth 2.0 Microsoft Exchange Email Connection
- Support for Existing MS OAuth Email Connection
- OAuth 2.0 MS Graph Email Connection
- Create a Connection for Office 365 GCC High
- Mobile Testing
- OrchestraCMS Testing
- Salesforce CPQ Testing
- ServiceMax Testing
- Skuid Testing
- Vlocity API Testing
- Webservices Testing
- Provar Manager
- How to Use Provar Manager
- Provar Manager Setup
- Provar Manager Integrations
- Release Management
- Test Management
- Test Operations
- Provar Manager and Provar Automation
- Setting Up a Connection to Provar Manager
- Object Mapping Between Automation and Manager
- How to Upload Test Plans, Test Plan Folders, Test Plan Instances, and Test Cases
- Provar Manager Filters
- Uploading Callable Test Cases in Provar Manager
- Uploading Test Steps in Provar Manager
- How to Know if a File in Automation is Linked in Test Manager
- Test Execution Reporting
- Metadata Coverage with Manager
- Provar Grid
- DevOps
- Introduction to Provar DevOps
- Introduction to Test Scheduling
- Apache Ant
- Configuration for Sending Emails via the Automation Command Line Interface
- Continuous Integration
- AutoRABIT Salesforce DevOps in Provar Test
- Azure DevOps
- Running a Provar CI Task in Azure DevOps Pipelines
- Configuring the Automation secrets password in Microsoft Azure Pipelines
- Parallel Execution in Microsoft Azure Pipelines using Multiple build.xml Files
- Parallel Execution in Microsoft Azure Pipelines using Targets
- Parallel execution in Microsoft Azure Pipelines using Test Plans
- Bitbucket Pipelines
- CircleCI
- Copado
- Docker
- Flosum
- Gearset
- GitHub Actions
- Integrating GitHub Actions CI to Run Automation CI Task
- Remote Trigger in GitHub Actions
- Parameterization using Environment Variables in GitHub Actions
- Parallel Execution in GitHub Actions using Multiple build.xml Files
- Parallel Execution in GitHub Actions using Targets
- Parallel Execution in GitHub Actions using Test Plan
- Parallel Execution in GitHub Actions using Job Matrix
- GitLab Continuous Integration
- Travis CI
- Jenkins
- Execution Environment Security Configuration
- Provar Jenkins Plugin
- Parallel Execution
- Running Provar on Linux
- Reporting
- Salesforce DX
- Git
- Version Control
- Salesforce Testing
- Recommended Practices
- Salesforce Connection Best Practices
- Improve Your Metadata Performance
- Java 21 Upgrade
- Testing Best Practices
- Automation Planning
- Supported Testing Phases
- Provar Naming Standards
- Test Case Design
- Create records via API
- Avoid using static values
- Abort Unused Test Sessions/Runs
- Avoid Metadata performance issues
- Increase auto-retry waits for steps using a global variable
- Create different page objects for different pages
- The Best Ways to Change Callable Test Case Locations
- Working with the .testProject file and .secrets file
- Best practices for the .provarCaches folder
- Best practices for .pageObject files
- Troubleshooting
- How to Use Keytool Command for Importing Certificates
- Installing Provar After Upgrading to macOS Catalina
- Browsers
- Configurations and Permissions
- Add Permissions to Edit Provar.ini File
- Configure Provar UI in High Resolution
- Enable Prompt to Choose Workspace
- Increase System Memory for Provar
- Refresh Org Cache Manually
- Show Hidden Provar Files on Mac
- Java Version Mismatch Error
- Unable to test cases, test suites, etc… from the Test Project Navigation sidebar
- Connections
- DevOps
- Error Messages
- Provar Manager 3.0 Install Error Resolution
- Provar Manager Test Case Upload Resolution
- Administrator has Blocked Access to Client
- JavascriptException: Javascript Error
- Resolving Failed to Create ChromeDriver Error
- Resolving Jenkins License Missing Error
- Resolving Metadata Timeout Errors
- Test Execution Fails – Firefox Not Installed
- Selenium 4 Upgrade
- Licensing, Installation and Firewalls
- Memory
- Test Builder and Test Cases
- Release Notes